The previous chapter discussed Inheritance in Python and the super() method usage. We also understood how Inheritance could help reuse the code instead of writing the same methods repeatedly. In this chapter, we will discuss the types of Inheritance in Python.
Types of Inheritances in Python
Based on the number of classes you have, Python has different kinds of Inheritance, as listed below.
- Single Inheritance
- Multiple Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
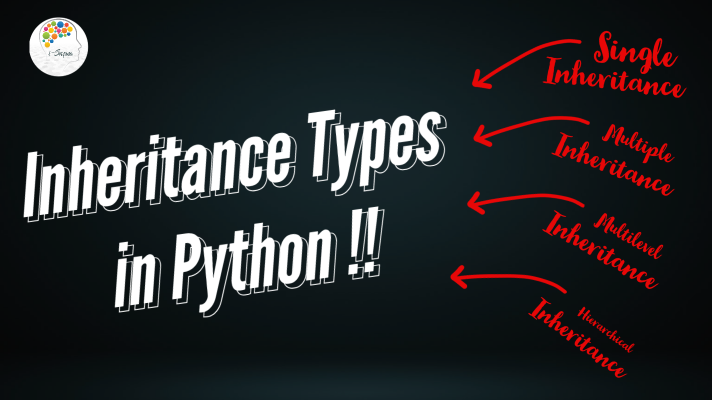
Single Inheritance
Single Inheritance occurs in a program only when one parent class gets inherited by one child class. Most of the programs we came across in the previous chapter have Single Inheritance. However, we will look at one more example program now.
class Teacher:
def method1(self):
print("This is method one")
class Child(Teacher):
def method2(self):
print("This is method two")
obj = Child()
obj.method1()
obj.method2()
Output
This is method one
This is method two
Multiple Inheritance
Multiple Inheritance is when a child class inherits more than one parent class, as shown in the program below.
class Teacher:
def method1(self):
print("This is method 1")
class Principal:
def method2(self):
print("This is method 2")
class Student(Teacher,Principal):
def method3(self):
print("This is method 3")
obj = Student()
obj.method1()
obj.method2()
obj.method3()
Output
This is method 1
This is method 2
This is method 3
You can see in the program above that we have two parent classes – Teacher and Principal. Both the parent classes are inherited by the child class, Student. When we created an object or instance for the Student class, we could access method1 and method2 from the parent classes.
Multilevel Inheritance
Multilevel Inheritance happens when a child class also becomes a parent for another class in a program.
#Multilevel Inheritance Example
class Father:
def method1(self):
print("This is method 1")
class Son(Father):
def method2(self):
print("This is method 2")
class Grandson(Son):
def method3(self):
print("This is method 3")
obj = Grandson()
obj.method1()
obj.method2()
obj.method3()
Output
This is method 1
This is method 2
This is method 3
You can see in the program above that the class Son inherited properties of the Father class. Similarly, the Grandson class also inherited properties of the Son class. But since the Son class has the properties of the Father class, the properties of both Father and Son classes become available to the Grandson class. However, the Grandson class has mentioned only the Son class in the declaration part.
Hierarchical Inheritance
Hierarchical Inheritance happens when several inheritances occur from the same parent or base class.
class Father:
def method1(self):
print("This is method 1")
class Son(Father):
def method2(self):
print("This is method 2")
class Daughter(Father):
def method3(self):
print("This is method 3")
s = Son()
d = Daughter()
s.method1()
s.method2()
d.method1()
d.method3()
Output
This is method 1
This is method 2
This is method 1
This is method 3
In the program above, you can notice that both the classes, Son and Daughter, inherit the properties from the Father class. And each child class was able to access only their methods and the parent class’s method.
Hybrid Inheritance
Hybrid Inheritance is when more than one type of Inheritance feature is mixed. In other words, it is a mixture of more than two or more different types of inheritances. I know you are confused right now. That’s why please refer to the image and the program below.
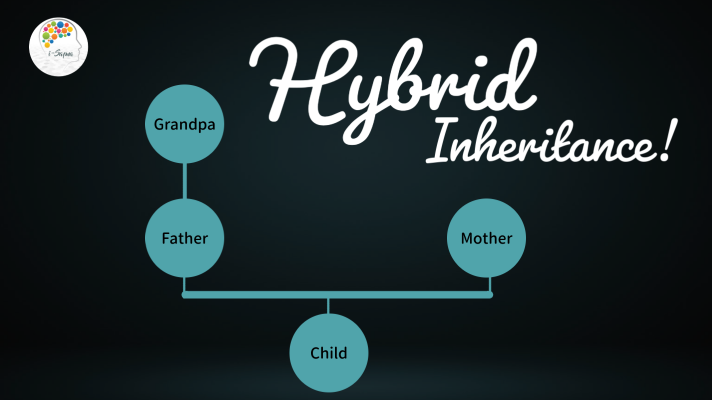
class Grandpa:
def method1(self):
print("This is method 1")
class Mother:
def method2(self):
print("This is method 2")
class Father(Grandpa):
def method3(self):
print("This is method 3")
class Child(Father, Mother):
def method4(self):
print("This is method 4")
obj1 = Father()
obj2 = Child()
print("*** Object 1 Methods ***")
obj1.method1()
obj1.method3()
print("*** Object 2 Methods ***")
obj2.method1()
obj2.method2()
obj2.method3()
obj2.method4()
Output
*** Object 1 Methods ***
This is method 1
This is method 3
*** Object 2 Methods ***
This is method 1
This is method 2
This is method 3
This is method 4
You can notice from the program above that we have several inheritances mixed into one program.
- Grandpa -> Father – Single Inheritance
- Grandpa -> Father -> Child – Multi-Level Inheritance
- Father -> Child <- Mother – Multiple Inheritance
Method Resolution Order (MRO) in Python
Any specified method or attribute is searched first in the current class in a multiple inheritance scenario. If it is not found there, the search will go on to parent classes in depth-first, left to right, without searching the same class more than once. This way of searching is called Method Resolution Order (MRO).
- The first rule in MRO is to look for a sub class before finding the base class.
- The second rule is that the order follows from left to right in the base classes when a class is inherited from several classes.
- The third and most important principle is that it will not go through any class twice and transits only once through each class.
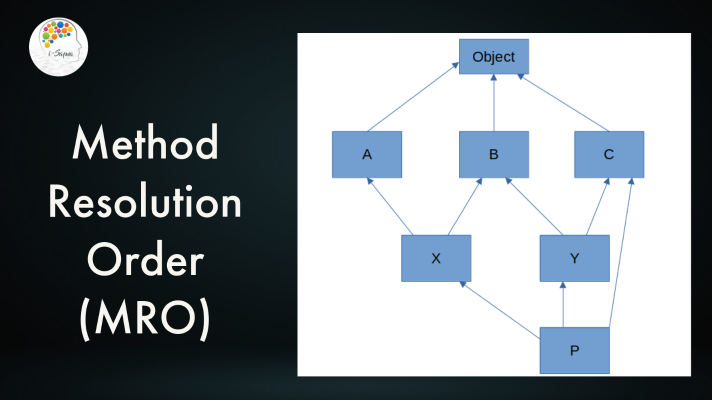
"""Python program to understand
Method Resolution Order"""
class A(object):
def method(self):
print("Method from Class A")
super().method()
class B(object):
def method(self):
print("Method from Class B")
super().method()
class C(object):
def method(self):
print("Method from Class C")
super().method()
class X(A,B):
def method(self):
print("Method from Class X")
super().method()
class Y(B,C):
def method(self):
print("Method from Class Y")
super().method()
class P(X,Y,C):
def method(self):
print("Method from Class P")
super().method()
obj = P()
obj.method()
Output
Method from Class P
Method from Class X
Method from Class A
Method from Class Y
Method from Class B
Method from Class C
Traceback (most recent call last):
File "/home/aatmann/Documents/iSapna/Python/InherPoly/mro.py", line 29, in <module>
obj.method()
File "/home/aatmann/Documents/iSapna/Python/InherPoly/mro.py", line 27, in method
super().method()
File "/home/aatmann/Documents/iSapna/Python/InherPoly/mro.py", line 19, in method
super().method()
File "/home/aatmann/Documents/iSapna/Python/InherPoly/mro.py", line 7, in method
super().method()
File "/home/aatmann/Documents/iSapna/Python/InherPoly/mro.py", line 23, in method
super().method()
File "/home/aatmann/Documents/iSapna/Python/InherPoly/mro.py", line 11, in method
super().method()
File "/home/aatmann/Documents/iSapna/Python/InherPoly/mro.py", line 15, in method
super().method()
AttributeError: 'super' object has no attribute 'method'
The above program has displayed a few errors, and that’s expected since the ‘super’ object has no attribute ‘method’. What’s important is to understand the flow followed through each class, which is P, X, A, Y, B, and C. Please refer to the image above to understand better.