In this post, we will discuss Files in Python. Files have always been an essential part of our lives, even before the digital age. We as humans always need files to store the information. For example, in a school, you might need files like an attendance register; in a company, you might need a file that logs the in and out time of the employees. Nowadays, we rely on the files on our computers. Though the files are digitized today, they still share a common characteristic with those from the olden days.
Yes, you got that right, storage of data in files. We used to store data in files in the olden days, and we still save data on the files on our computers now. And using Python, you can perform a lot of operations on Files. You can automate many manual tasks using Python that are performed on files and much more.
Link to all chapters of our Python tutorial series: Learn Python
Types of Files in Python
We have two kinds of files in Python – Text files and Binary files.
The text files store the data in the form of characters. For example, if we store the name of a team member, Shabari and his salary of 80000, it will be stored as characters in the file. We use text files typically to store the strings or characters.
In Binary files, the data is stored in the form of bytes. For example, an integer is stored in 8 bytes and a character in the form of a byte. The developers retrieve data in the form of bytes in binary files.
You can store video, audio, images and text in binary files. For instance, if you look at an image file, you will find many pixels displayed on the screen. You can represent each pixel either by 1 or 0 and eventually save them as binary files.
How to open files in Python?
Using Python, you can use the open() function to open a file. Please find below its format.
file1 = open(“name_of_file”, “mode_of_file”, “buffering”
- In the format share above, ‘file1‘ is the file handler or the variable to which we assign the file while opening it. The ‘name_of_file‘ refers to the file’s actual name, including the format. For example, ‘logfile.txt’.
- The ‘mode_of_file’ refers to the reading, writing, or other modes you can use to open the file. If you open a file in reading mode, you can only read the file, and you cannot write the file. Please refer to the table below to know all the file opening modes in Python.
- Buffering is an optional integer used to set the buffer’s size in a file. A buffer means a temporary block of memory. In the text mode, you can pass 1 as buffering to retrieve one line of data at a time from the file. In binary mode, you can use 0 as buffering to represent no buffering.
File mode | Description |
r | Used for reading data from file. |
w | Used for writing data into file. If you write data on a non-empty file, the existing data gets deleted, and the new data gets added. |
a | Used for appending data to the file. It adds the data at the end of the current data in the file. |
r+ | Used for reading and writing data in the file. The old data does not get deleted. |
w+ | Used for reading and writing data in the file. The old data gets deleted in this mode. |
a+ | Used for appending and reading data of a file. If the file is not present, it adds a new file for writing and reading. |
x | Used for creating file in an exclusive creation mode. The file creation operation fails if the fail is present already. |
Based on the information available above, if you want to open a file ‘somefile.txt’ in reading mode, you’d need to do that as shown below.
f = open(‘somefile.txt’,’r’)
How to close files in Python?
We can close the files in Python using the close() method. If a file is opened using the open() method, it is crucial to close it using the close() method. Otherwise, the file can still use the resources and cause issues related to the memory. You can find its format below.
f.close() #format to close the file
Let us look at a program now to put all our learnings into practice.
#Python program to create a file,
# write data into the file and then close the file.
f = open("myfirstfile.txt","w")
#add some characters from the keyboard
input_from_keyboard = input("Please enter some text: ")
#adding input to the file
f.write(input_from_keyboard)
#close the file
f.close()
Console Screen:
Please enter some text: Hello file world!

In the above program, we created a file, ‘myfirstfile.txt‘ in write mode and added input from the keyboard into the file. We also made sure to close the file at the end of the program.
Let us now look at another program that can read the contents from the same file, ‘myfirstfile.txt‘
#Python program to read from a file
f = open('myfirstfile.txt','r') #opening file in read mode
text = f.read() #reads all characters from the file
print(text) #displays the strings from the file on the screen
f.close() #closing the file
Output:
Hello file world!
In the above program, we opened the file, ‘myfirstfile.txt‘ in read mode. After reading the strings from the file using the read() method, we printed the same on the screen. You can notice in the output above that it printed the exact string we had provided as input in the first program of this post. It is evident from the programs above that we can use the methods write() and read() to write and read data in files, respectively.
How to check whether a file is present using Python?
We can use the isfile() method from the ‘os‘ module to know whether a file is present or not in a directory. Let us now look at a program to understand how we can implement the same.
#Python program to check if the file is present in the directory
import os, sys
#get the file name from the user
file_name = input("Please enter name of the file: ")
if os.path.isfile(file_name):
f = open(file_name,'r')
else:
print("The file '{}' is not available in this directory".format(file_name))
sys.exit() #exit from the program
#if file is present, print the content
text_in_file = f.read()
print("The text below is available in the given file")
print(text_in_file)
f.close() #closing the file
Output when the filename exists:
Please enter name of the file: myfirstfile.txt
The text below is available in the given file
Hello file world!
Output when the filename doesn't exist:
Please enter name of the file: test
The file 'test' is not available in this directory
We imported the ‘os‘ and ‘sys‘ modules in the program above in the first line. Using the isfile() method, we checked if the entered file was available in the directory. If the file is available, the console prints the file’s contents in the output. Suppose it is unavailable; the console prints that the file is not available in this directory and exits from the program execution. You can use this code to print even the contents of another python program.
How to count the number of characters, words, and lines of a file through a Python program?
We can count the number of characters, words, and lines of a file through a Python program. For testing purposes, we created a text file called ‘examplefile.txt‘ and added the content below.
iPhone is an amazing smartphone
Apple is best at amazing its customers with new features every year
It really takes a lot of hardwork to continue the momentum
The file above has three lines, but we might not be able to count the number of words and characters without putting additional effort manually. Let us now look at a program shown below, which can give us the exact count of the words, lines, and characters in the file, ‘examplefile.txt‘.
#Python program to count the number of characters, lines and words
#in a file
import os,sys
file_name = input("Enter name of file: ")
if os.path.isfile(file_name):
f = open(file_name, 'r')
else:
print('The "{}" file does not exist in this directory'.format(file_name))
sys.exit()
#initialize all counters to zero
line_count = word_count = char_count = 0
#read line after line from the file
for every_line in f:
words = every_line.split()
line_count += 1
word_count += len(words)
char_count += len(every_line)
print("Number of lines in the file: ",line_count)
print("Number of words in the file: ",word_count)
print("Number of characters in the file: ",char_count)
#closing the file
f.close()
Output:
Enter name of file: examplefile.txt
Number of lines in the file: 3
Number of words in the file: 28
Number of characters in the file: 158
How to work with Binary files in Python?
As mentioned at the beginning of this post, we can use the Binary files with data in bytes to deal with videos, audio, images and text data. Let us now look at a program that can copy the data from one image to another by copying the former’s binary data.
I saved the below image as laptop.jpg in my current directory.
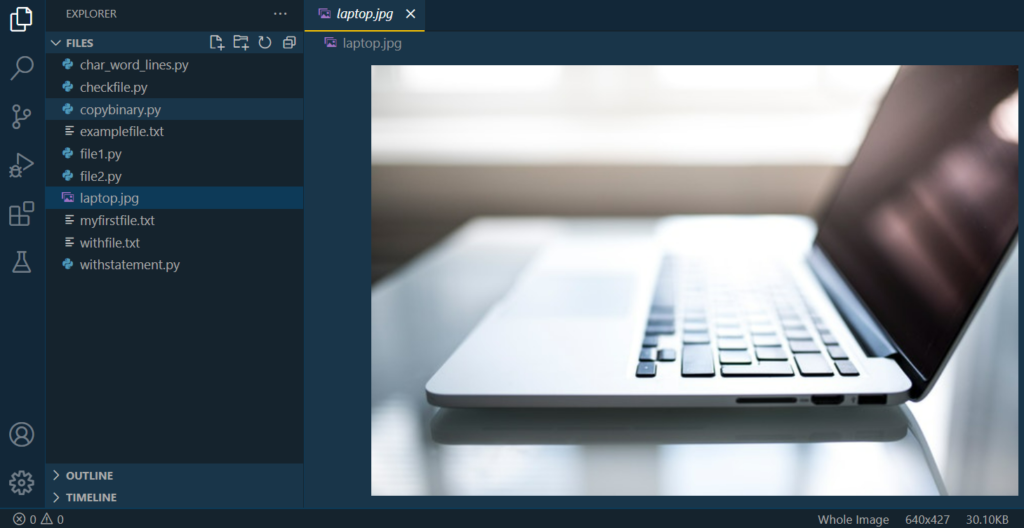
Now let us create a copy of it in the same directory using the Python code.
#Python program to create a copy of an image using code
#open the files in binary mode
file1 = open('laptop.jpg','rb')
file2 = open('copyoflaptop.jpg','wb')
#copy data from file1 to file2
data_from_file1 = file1.read()
file2.write(data_from_file1)
#close the files
file1.close()
file2.close()
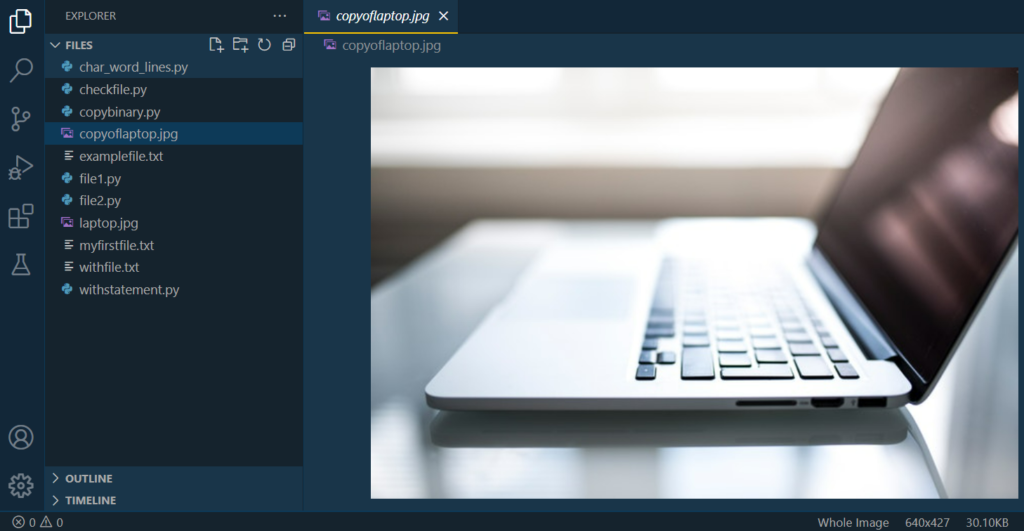
You can notice from the screenshot below that a new image with the name ‘copyoflaptop.jpg‘ is available now in the current directory.
Since we had to deal with the binary data in this program, we used the ‘rb‘ and ‘wb‘ modes to read and write the files in binary mode, respectively.
The with statement to work with files in Python
You can use the ‘with‘ statement to open a file in Python. And it has an advantage over opening the files in the regular mode. You need not mention the close() method when you use a ‘with‘ statement in Python. The files get closed automatically.
Let us now look at a program to understand how we can open a file and write data using the with statement for files in Python.
#Python program to show the usage of with statement
with open('withfile.txt','w') as file1:
file1.write('Python is amazing\n')
file1.write('I will learn and master this language')
Running the program above created a new file in the same directory with the strings mentioned in the screenshot below.

Similarly, you can use the with statement to open the files in read mode and get the data. Try coding that program yourself to test your programming skills.